list of some daily uses WordPress hooks with example
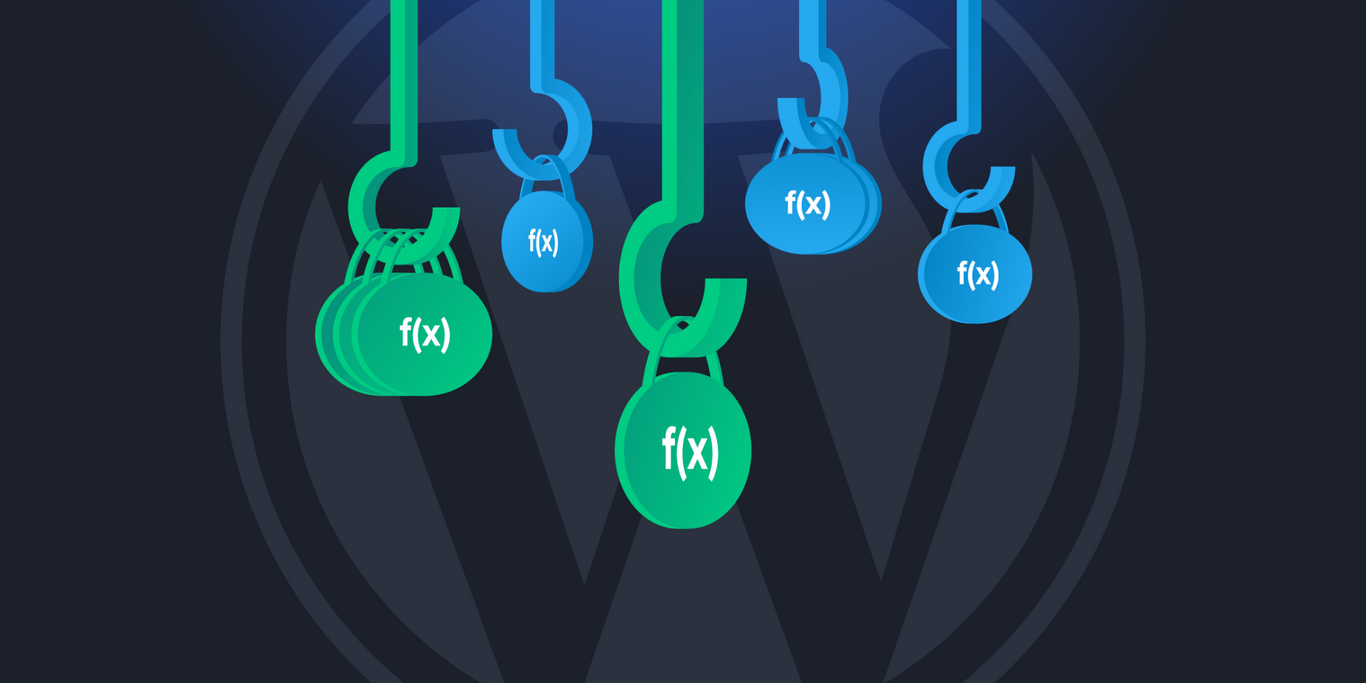
-
wp_head Action Hook:
- Description: Used to inject code into the <head> section of the HTML document.
- Example:
function custom_code_in_head() { echo '<meta name="description" content="Custom description">'; } add_action('wp_head', 'custom_code_in_head');
-
wp_footer Action Hook:
- Description: Allows insertion of code just before the closing </body> tag.
- Example:
function custom_code_in_footer()
{ echo '<script>alert("Hello, World!");</script>'; }
add_action('wp_footer', 'custom_code_in_footer');
-
init Action Hook:
- Description: Used for initializing code or actions.
- Example:
function custom_init_function() { // Your initialization code here } add_action('init', 'custom_init_function');
2. Filter Hooks:
the_content Filter Hook:
-
- Description: Allows modification of the content before it is displayed.
- Example:
function custom_content_filter($content) { return $content . '<p>Custom content added.</p>'; }
add_filter('the_content', 'custom_content_filter');
-
the_title Filter Hook:
- Description: Used to modify the post/page title before it is displayed.
- Example:
function custom_title_filter($title)
{ return 'Custom Prefix: ' . $title; }
add_filter('the_title', 'custom_title_filter');
-
excerpt_length Filter Hook:
- Description: Adjusts the length of the excerpt.
- Example:
function custom_excerpt_length($length)
{ return 20; // Set the desired length }
add_filter('excerpt_length', 'custom_excerpt_length');
admin_menu Action Hook:
- Description: Used to add menu items to the WordPress admin menu.
- Example:
function add_custom_menu()
{ add_menu_page('Custom Page', 'Custom Menu', 'manage_options', 'custom-page', 'custom_page_callback'); }
add_action('admin_menu', 'add_custom_menu'); function custom_page_callback() { // Content for the custom admin page }
widgets_init Action Hook:
- Description: Enables the registration of custom widgets.
- Example:
function register_custom_widget() { register_widget('Custom_Widget_Class'); }
add_action('widgets_init', 'register_custom_widget'); class Custom_Widget_Class extends WP_Widget
{ // Custom widget implementation }
the_excerpt Filter Hook:
- Description: Modifies the excerpt content before it is displayed.
- Example:
function custom_excerpt_filter($excerpt)
{
return $excerpt . ' <a href="' . get_permalink() . '">Read more</a>';
}
add_filter('the_excerpt', 'custom_excerpt_filter');
login_headerurl Filter Hook:
Description: Modifies the login page header URL.
Example:
function custom_login_url() {
return home_url('/');
}
add_filter('login_headerurl', 'custom_login_url');
wp_nav_menu_items
Filter Hook:Description: Adds custom items to the navigation menu.
Example:
function add_custom_menu_item($items, $args) {
if ($args->theme_location == 'primary') {
$items .= '<li><a href="#">Custom Link</a></li>';
}
return $items;
}
add_filter('wp_nav_menu_items', 'add_custom_menu_item', 10, 2);
-
save_post Action Hook:
Description: Triggered after a post or page is saved in the database.
Example:
function custom_save_post_callback($post_id, $post, $update) {
// Custom logic to be executed after post/page is saved
}
add_action('save_post', 'custom_save_post_callback', 10, 3);
wp_enqueue_scripts Action Hook:
Description: Used to enqueue scripts and styles on the front end.
Example:
function enqueue_custom_scripts() {
wp_enqueue_script('custom-script', get_template_directory_uri() . '/js/custom-script.js', array('jquery'), '1.0', true);
}
add_action('wp_enqueue_scripts', 'enqueue_custom_scripts');
-
the_title Filter Hook:
Description: Modifies the post or page title before it is displayed.
Example:
function custom_title_filter($title) {
return 'Custom Prefix: ' . $title;
}
add_filter('the_title', 'custom_title_filter');
excerpt_length Filter Hook:
Description: Modifies the number of words in the excerpt.
Example:
function custom_excerpt_length($length)
{ return 20; // Set the custom excerpt length }
add_filter('excerpt_length', 'custom_excerpt_length');
body_class Filter Hook:
Description: Adds custom classes to the body element.
Example:function add_custom_body_class($classes) {
$classes[] = 'custom-class';
return $classes;
}
add_filter('body_class', 'add_custom_body_class');
Sponsored
Search
Sponsored
Sponsored
Categories
- Web Development
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film
- Fitness
- Food
- Games
- Gardening
- Health
- Home
- Literature
- Music
- Networking
- Other
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
Read More
JioSphere vs Bitcoin: Understanding the Difference Between Immersive Tech and Digital Currency
Technology has brought countless innovations to our lives, with two of the most exciting trends...
Toulene Market Size, Competitive Landscape, Regional Outlook and COVID-19 Impact Analysis 2023
Introduction:
Toluene is an aromatic hydrocarbon commonly derived from petroleum and coal tar....
Mobile Optimization for SEO: Best Practices
Mobile optimization is an indispensable aspect of SEO (Search Engine Optimization). It plays a...
How a Web Developer Can Take Interest in Their Own Work: Unlocking Passion and Motivation
In the fast-paced world of web development, it's easy to get caught up in the grind of coding,...
Spunbond Nonwoven Market 2023 COVID-19 Impact, Share, Trend, Segmentation and Forecast to 2032
Introduction:
Spunbond nonwoven fabric is a versatile material widely used in various industries...