Curl vs wp_get_remote_post in wordpress
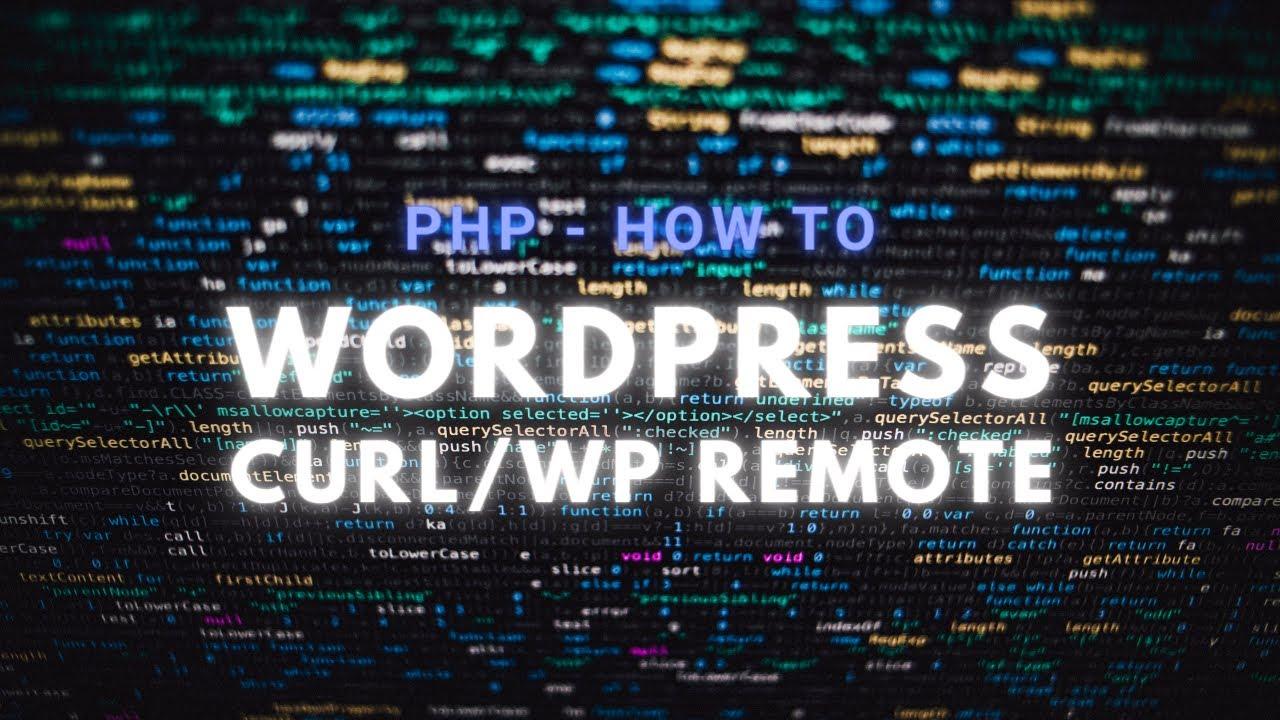
curl
and wp_remote_post
are both methods used in WordPress for making HTTP requests, but they have different use cases and implementations.
-
cURL (Client for URLs):
- Usage:
curl
is a command-line tool and library for making HTTP requests. In PHP, you can use thecurl
library functions to make HTTP requests. - Flexibility:
curl
is a more general-purpose tool that can be used for various protocols, not just HTTP. It provides a wide range of options and settings for making requests.
- Usage:
Code : $ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://example.com/api');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$response = curl_exec($ch);
curl_close($ch);-
wp_remote_post
:- Usage:
wp_remote_post
is a WordPress function specifically designed for making HTTP POST requests. - Integration: It is part of the HTTP API provided by WordPress, making it more integrated and easier to use within WordPress plugins or themes.
- Security and Context:
wp_remote_post
incorporates WordPress security and context features, such as nonce verification and cookie handling.$response = wp_remote_post('https://example.com/api', array(
'body' => array('key1' => 'value1', 'key2' => 'value2'),
)); -
Choosing Between
curl
andwp_remote_post
in WordPress:- If you are working within the WordPress environment and specifically need to make a POST request,
wp_remote_post
is often a more convenient and integrated option. - If you have more complex requirements, need to make requests using different HTTP methods, or are not within the WordPress context, using the
curl
library functions might be more appropriate.
In general,
wp_remote_post
is favored when working within the WordPress ecosystem due to its simplicity and integration, whilecurl
provides more flexibility for broader use cases. - If you are working within the WordPress environment and specifically need to make a POST request,
- Usage:
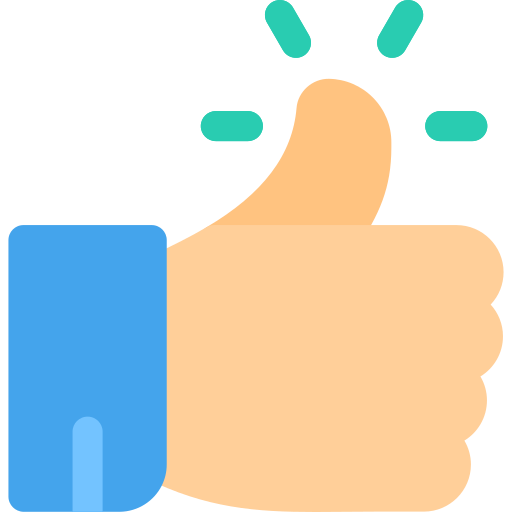
Sponsored
Search
Sponsored
Sponsored
Categories
- Web Development
- Art
- Causes
- Crafts
- Dance
- Drinks
- Film
- Fitness
- Food
- Games
- Gardening
- Health
- Home
- Literature
- Music
- Networking
- Other
- Party
- Religion
- Shopping
- Sports
- Theater
- Wellness
Read More
True Indian Village Fitness: A Natural Way to Stay Healthy
In the heart of India’s villages lies a timeless secret to fitness. Without gyms, gadgets,...
How to Connect a WordPress Website with Hardware
Explain that, while WordPress is traditionally used for web content, it can communicate with...
INS Jatayu
’INS Jatayu’ in Lakshadweep for Better Coverage of Indian Ocean
To bolster its...
Some Hidden WordPress Features You Must Know for Better Website Management
WordPress is the world's most popular content management system, powering over 40% of all...
WordPress Query Optimization: Enhancing Performance and Reducing Load Time
WordPress relies heavily on database queries to fetch and display content dynamically. While its...